Get domain, path, protocol and get parameters in Django template
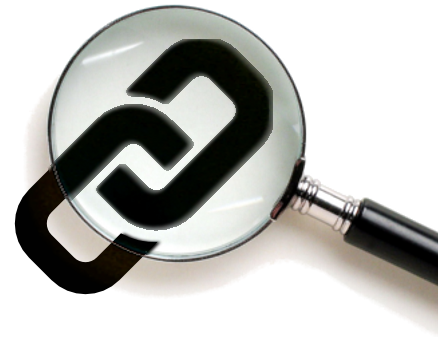
If you are a Django web developer you surely have found the need to use the sites domain name or absolute URL in a template. Django has a lot of functions and META keywords to do just that, but the features are spread out and lack a common interface. So, we have made a small tool/library to simplify the process of getting what you need from the complex URL/URI schema.
What the fuzz?
So what exactly is the problem?Why would you need the full URL? And why not just always use relative paths, no domain needed?
Well first, say you want to share the current page on a social network. And you don’t want bloated prefab share buttons but use static share buttons. You will need to get the current URL in you template code to use in the share link. And not just the relative path, but the full URL, say: http://example.com/news/2014/11/awesome-news/ instead of /news/2014/11/awesome-news/. Getting that URL is surprisingly poorly documented.
Or say you want to send your users and email with your brand image in the header. If you simple use
{% static "my_app/myexample.jpg" %}
you’ll end up with a broken image if the user views the email outside of your web page. Why, because in their web mail viewer or native app the domain name is unkown. Solution? Add the domain name in front of the image path.
So our little library should make it easy to get an URL in it’s entirety or just some parts of it. Say the user is looking at the URL: http://localhost:8000/news/2014/?sort=ascending#item2
Using our library you can easily obtain the different parts such as:
- protocol:
http://
- domain:
localhost:8000
- port:
8000
- path:
/news/2014/
- query:
sort=ascending
- full_url:
/news/2014/?sort=ascending
- absolute_uri:
http://localhost:8000/news/2014/?sort=ascending
To actually get these URL parts install the plugin and use either the context manager method or add the variables to the context yourself. For the rest of the text, we’ll assume you are using the context manager.
Full URL
If you want to share the current page you probably want to have access to the full URL in your template. If you want the protocol, domain and path without get parameters use:
{{url_parts.protocol}}{{url_parts.domain}}{{url_path}}
If you also want the get parameters you can use the simpler function:
{{url_parts.absolute_uri}}
Doing the latter is easy in Vanilla Django using “build_absolute_uri”. The former requires you to add the domain and path after “http://” or “https://” depending whether the request is secured or not.
(re)direct your users after login Note that if you want to use the current page to create a login button that redirects after authentication you will want to use the “full_url”. Your template code should look like:
{% url "login" %}?next={{url_parts.full_url}}
The default Django auth login view handles the redirect for you using the “next” get parameter.
Domain or host name
If you use a rendered template outside of your website you can’t just use relative paths. So you’ll need the domain name of the current site. The site framework does this for you, but it’s a hassle and prone to errors because configuration is stored in the database. To get the domain name in the template use:
{{url_parts.domain}}
Simple enough right? So if you for example use this to add an image in your outgoing emails, you’ll want to do:
<img src="http://{{url_parts.domain}}{% static "img/my-brand-logo.png" %}" alt="My brand logo" />
Protocol, port, query
Finally the infrequently required URL parts. In some rare cases you might want to extract some very specific information from the URL. You can obtain the protocol (“http://” or “https://”) by:
{{url_parts.protocol}}
The port can be extruded from the request is certain situations (not all) by:
{{url_parts.port}}
Finally you might just want to get the query part of the URL, you know, that part behind the “?” with the get parameters:
{{url_parts.query}}
Convenient bits and pieces
So, what we aimed to create was a small tool that makes using URL bits and pieces a bit more convenient than the Django way. We use it on our site for static share links, login redirects and email images. We hope you will be able to use it as well. And please let us know if you would like a feature or found a bug.
Related
This post is part of the project CodeCook.io
- Next post: Django Markdownify
- Previous post: Static social share buttons