Custom component highlight bookmarklet
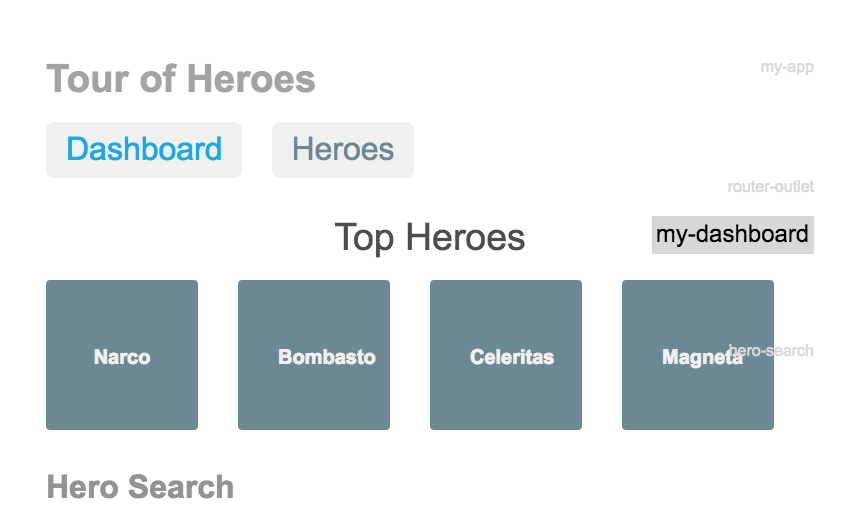
A good way to get a feel for a web application is to first figure out the component hierarchy. To aid in understanding the component structure we will create a simple bookmarklet that highlights custom components.
Understanding an App is easier once you have a mental map how pages are structured. Because it shows how the (hopefully) flat component structure and actual components work together. We'll look how we can identify app components structure by adding some custom styling to all none standard HTML tags using a bookmarklet.
The end result is a bookmarklet that shows all components on the current page:
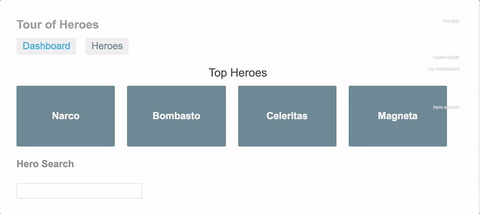
Try it out yourself or read on to see how it works.
Highlight app component HTML tags
The goal is to quickly see how a web app is composed by showing all web components.
<body>
<my-app>
<div>
<my-list>
<my-item></my-item>
<my-item></my-item>
</my-list>
</div>
</my-app>
</body>
Say you have the basic application structure from above.
We will look how we can determine and style the my-app
, my-list
and my-item
components so you can easily identify them as a developer.
In order to use the technique as widely as possible we will look at a library agnostic solution. That means we can't hook into the your view library of choice to determine all components. We are stuck with what we can gather from the DOM. Luckily we can do this because custom components should not overlap with any standard HTML tags. So we can just select all none standard tags.
This approach means that:
- Only components that have custom HTML tags in the final page are shown, so Angular components are selected, React components are not.
- We have an admittedly hacky approach to do this, but hey, as long as it works, right :).
Selecting components
The first step in showing new HTML tags is finding them on the current page. Luckily these custom elements have one very clear property, they are not a standard HTML tag.
A quick look at MDN yields the following list:
knownTags = ['address', 'article', 'aside', 'footer', 'h1','h2','h3','h4','h5','h6', 'header', 'hgroup', 'nav', 'section', 'dd', 'div', 'dl', 'dt', 'figcaption', 'figure', 'hr', 'li', 'main', 'ol', 'p', 'pre', 'ul', 'a', 'abbr', 'b', 'bdi', 'bdo', 'br', 'cite', 'code', 'data', 'dfn', 'em', 'i', 'kbd', 'mark', 'q', 'rp', 'rt', 'rtc', 'ruby', 's', 'samp', 'small', 'span', 'strong', 'sub', 'sup', 'time', 'u', 'var', 'wbr','caption', 'col', 'colgroup', 'table', 'tbody', 'td', 'tfoot', 'th', 'thead', 'tr', 'button', 'datalist', 'fieldset', 'form', 'input', 'label', 'legend', 'meter', 'optgroup', 'option', 'output', 'progress', 'select', 'textarea' ]
We want to select all elements that do not have these tags. For that we can use the CSS not selector like:
:not( address ):not( article ) ...
We create the selector and find nodes using:
notCss = knownTags.map(tag => `:not( ${ tag } )`).join('')
nodes = document.querySelectorAll(`body ${ notCss }`)
The actual full CSS selector is very long:
:not( address ):not( article ):not( aside ):not( footer ):not( h1 ):not( h2 ):not( h3 ):not( h4 ):not( h5 ):not( h6 ):not( header ):not( hgroup ):not( nav ):not( section ):not( dd ):not( div ):not( dl ):not( dt ):not( figcaption ):not( figure ):not( hr ):not( li ):not( main ):not( ol ):not( p ):not( pre ):not( ul ):not( a ):not( abbr ):not( b ):not( bdi ):not( bdo ):not( br ):not( cite ):not( code ):not( data ):not( dfn ):not( em ):not( i ):not( kbd ):not( mark ):not( q ):not( rp ):not( rt ):not( rtc ):not( ruby ):not( s ):not( samp ):not( small ):not( span ):not( strong ):not( sub ):not( sup ):not( time ):not( u ):not( var ):not( wbr ):not( caption ):not( col ):not( colgroup ):not( table ):not( tbody ):not( td ):not( tfoot ):not( th ):not( thead ):not( tr ):not( button ):not( datalist ):not( fieldset ):not( form ):not( input ):not( label ):not( legend ):not( meter ):not( optgroup ):not( option ):not( output ):not( progress ):not( select ):not( textarea )
Next up is actually highlighting these non standard elements in the DOM.
Highlighting
To highlight the list of DOM nodes we'll append a absolute positioned styled element. Nothing to fancy.
One thing of note is using adding the CSS to the first style sheet we encounter. Of course, we could also inline the CSS, but this just seemed a bit nicer.
The result is as follows:
nodesArray.map( node => {
const outer = document.createElement('div');
outer.className='debug-wrapper';
const inner = document.createElement('div');
inner.innerHTML = node.nodeName.toLowerCase();
inner.className='debug-component';
node.prepend(outer);
outer.appendChild(inner);
});
const rules = [`
.debug-wrapper {
position: relative;
}`,`
.debug-component {
position: absolute;
right: 0;
z-index: 777;
color: lightgray;
font-size: 8px;
}`,`
.debug-component:hover {
color: black;
background-color: lightgray;
font-size: 12px;
padding: 0.2em;
}`];
const sheet = window.document.styleSheets[0];
rules.map(rule => sheet.insertRule(rule, sheet.cssRules && sheet.cssRules.length || 0) );
For the complete code see the GitHub project.
Creating a bookmarklet
Now one way to apply this code to the current page is to drop in in the browser console. That however is a bit tedious, so let us look another deployment method: a bookmarklet.
Creating a bookmarklet is surprisingly simple, stupidly simple. You just dump your entire script inside an anchor tag like:
<a href="javascript:<my-custom-code>">My custom code is executed if dragged to bookmarks list and pressed</a>
Highlighting bookmark
Applying the custom tag highlighting code to a bookmarklet is done by creating an anchor tag with the following href
<a href="javascript:'use strict';(function(){var knownTags=['address','article','aside','footer','h1','h2','h3','h4','h5','h6','header','hgroup','nav','section','dd','div','dl','dt','figcaption','figure','hr','li','main','ol','p','pre','ul','a','abbr','b','bdi','bdo','br','cite','code','data','dfn','em','i','kbd','mark','q','rp','rt','rtc','ruby','s','samp','small','span','strong','sub','sup','time','u','var','wbr','caption','col','colgroup','table','tbody','td','tfoot','th','thead','tr','button','datalist','fieldset','form','input','label','legend','meter','optgroup','option','output','progress','select','textarea'];var notCss=knownTags.map(function(tag){return':not( '+tag+' )';}).join('');var nodes=document.querySelectorAll('body '+notCss);var nodesArray=Array.prototype.slice.call(nodes);nodesArray.map(function(node){var outer=document.createElement('div');outer.className='debug-wrapper';var inner=document.createElement('div');inner.innerHTML=node.nodeName.toLowerCase();inner.className='debug-component';node.prepend(outer);outer.appendChild(inner);});var rules=['\n .debug-wrapper {\n position: relative;\n }','\n .debug-component {\n position: absolute;\n right: 0;\n z-index: 777;\n color: lightgray;\n font-size: 8px;\n }','\n .debug-component:hover {\n color: black;\n background-color: lightgray;\n font-size: 12px;\n padding: 0.2em;\n }'];var sheet=window.document.styleSheets[0];rules.map(function(rule){return sheet.insertRule(rule,sheet.cssRules&&sheet.cssRules.length||0);});})();">Highlight custom components Bookmarklet (Drag and drop this to you bookmarks list)</a>
The resulting link can be added to your bookmarks bar and used to highlight custom components on any page:
Highlight custom components Bookmarklet (Drag and drop this to you bookmarks list)
Using the bookmarklet is remarkably easy, just drag and drop the link above to your browsers bookmarks list.
Next, navigate to a page with custom tags (An old Angular App for example) and press the bookmarklet while you are on the page.
For example try the Angular material site
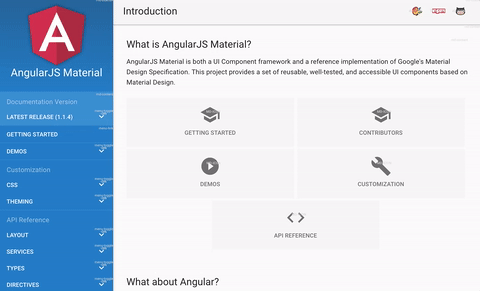